An alarm clock is always handy to alert us whenever we sleep, take a short nap, or to remind us about the work, we always get oblivious about.
The objective of our project is to implement an alarm clock using Python. Python consists of some very innovative libraries such as datetime and tkinter which help us to build the project using the current date and time as well as to provide a user interface to set the alarm according to the requirement in 24-hour format.
Prerequisites
This project requires good knowledge of Python and GUI. Python when combined with Tkinter provides a fast and easy way to create GUI applications. Tkinter provides a powerful object-oriented interface to the Tk GUI toolkit. All the modules used need not be downloaded beforehand like the other libraries like NumPy, thus this project will be user friendly and accessible in any virtual environment used for python programming.
Let’s start
First, let’s check the steps to build an Alarm Clock program in Python:
- Installing and Importing all the libraries and modules required
- Putting forward a while loop which takes the argument of the time, the user want his to set the alarm on and automatically breaks when the time is up, with sound.
- Create a display window for user input.
So that is basically what we will do in this Python project. Let’s start.
1. First, we import all the necessary libraries and modules:
#Importing all the necessary libraries to form the alarm clock:
from tkinter import *
import datetime
import time
import winsound
2. Create a while loop:
def alarm(set_alarm_timer):
while True:
time.sleep(1)
current_time = datetime.datetime.now()
now = current_time.strftime(“%H:%M:%S”)
date = current_time.strftime(“%d/%m/%Y”)
print(“The Set Date is:”,date)
print(now)
if now == set_alarm_timer:
print(“Time to Wake up”)
winsound.PlaySound(“sound.wav”,winsound.SND_ASYNC)
break
def actual_time():
set_alarm_timer = f”{hour.get()}:{min.get()}:{sec.get()}”
alarm(set_alarm_timer)
3. Creating GUI using tkinter:
clock = Tk()
clock.title(“Alarm Clock”)
clock.geometry(“400×200”)
time_format=Label(clock, text= “Enter time in 24 hour format!”, fg=”red”,bg=”yellow”,font=”Arial”).place(x=60,y=120)
addTime = Label(clock,text = “Hour Min Sec”,font=60).place(x = 110)
setYourAlarm = Label(clock,text = “When to wake you up”,fg=”blue”,font=(“Helevetica”,7,”bold”)).place(x=0, y=29)
# The Variables we require to set the alarm(initialization):
hour = StringVar()
min = StringVar()
sec = StringVar()
#Time required to set the alarm clock:
hourTime = Entry (clock,textvariable = hour, bg = “pink”, width = 15).place(x=110,y=30)
minTime= Entry(clock,textvariable = min,bg = “pink”,width = 15).place(x=150,y=30)
secTime = Entry(clock,textvariable = sec,bg = “pink”,width = 15).place(x=200,y=30)
#To take the time input by user:
submit = Button(clock,text = “Set Alarm”,fg=”red”,width = 10,command = actual_time).place(x =110,y=70)
clock.mainloop()
Result time!
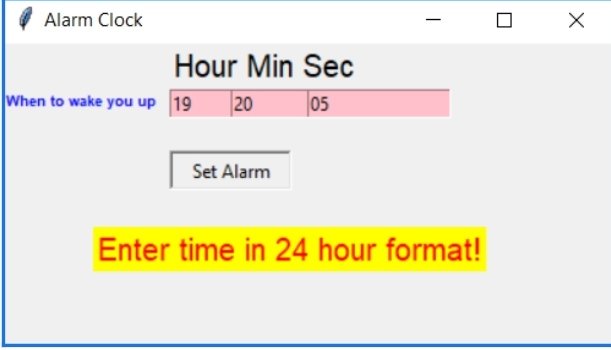
Summary
With this project in Python, we have successfully made the Alarm Clock. We used the popular GUI library for rendering graphics on a display window. We learned how to extract the current time from the computer and to use it for manipulation using the DateTime library. This way we can set an alarm in the computer interface using python programming which rings with the default machine sound for Windows.
This post was curated with the help of the highly skilled Vaishali Anand, a Python expert who is interning under us.